wtyczki — najlepsze podejście do zmiany obrazu tła telefonu komórkowego i dodania jego klasy
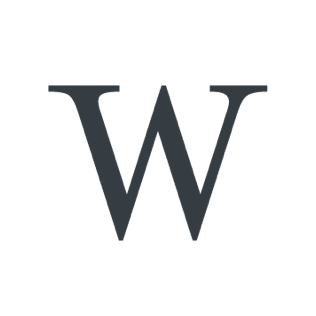
- przedłużyłem
core/group
block, aby dodać inny obraz tła podczas oglądania w mniejszym widoku. - Chciałbym wiedzieć, jakie będzie najlepsze podejście do dodania tutaj nazwy klasy, co zrobiłem w moim kodzie lub czegoś innego. Wątpię też, czy zdecyduję się zmienić obraz za pomocą CSS, to dla wszystkich grup na pojedynczej stronie obraz tła na urządzeniu mobilnym będzie taki sam i jeśli zdecyduję się to zrobić za pomocą JS, wówczas zostanie uruchomione tylko odświeżenie strony.
/**
* WordPress dependencies
*/
const { addFilter } = wp.hooks;
const { InspectorControls } = wp.blockEditor;
const { PanelBody } = wp.components;
const { createHigherOrderComponent } = wp.compose;
const { MediaUpload, MediaUploadCheck } = wp.blockEditor;
const { Fragment } = wp.element;
const { __ } = wp.i18n;
/**
* Adds the mobileBackgroundImage attribute to the group block settings.
*
* @param {Object} settings - The block settings.
* @return {Object} - The updated block settings.
*/
function addMobileBackgroundImageAttribute( settings ) {
if ( 'core/group' !== settings.name ) {
return settings; // Return settings even if the block name doesn't match
}
settings.attributes = {
...settings.attributes,
mobileBackgroundImage: {
type: 'string',
default: '',
},
};
return settings;
}
addFilter( 'blocks.registerBlockType', 'addMobileBackgroundImageAttribute', addMobileBackgroundImageAttribute );
/**
* Higher Order Component to add class name and inspector controls to core/group block.
* This HOC adds a mobile background image control to the core/group block.
*
* @param {Function} BlockEdit - Original component.
* @return {Function} - New component.
*/
const withClassNameAndInspectorControls = createHigherOrderComponent( ( BlockEdit ) => {
return ( props ) => {
const { name, attributes, setAttributes } = props;
if ( 'core/group' !== name ) {
return <BlockEdit { ...props } />;
}
const oldClassList = attributes.className ? attributes.className : '';
const removeClass = oldClassList?.replace( 'has-mobile-background-image', '' );
return (
<>
<BlockEdit { ...props } />
<InspectorControls group="styles">
<PanelBody title={ __( 'Mobile Background Image', 'features' ) }>
<MediaUploadCheck>
<MediaUpload
onSelect={ ( media ) => {
setAttributes( { mobileBackgroundImage: media.url, className: oldClassList + ' has-mobile-background-image' } );
} }
type="image"
value={ attributes.mobileBackgroundImage }
render={ ( { open } ) => (
<>
<button className="button button-large" onClick={ open }>
{ attributes.mobileBackgroundImage ? __( 'Change Image', 'features' ) : __( 'Select Image', 'features' ) }
</button>
{ attributes.mobileBackgroundImage && (
<>
<img src={ attributes.mobileBackgroundImage } alt={ __( 'Mobile Background', 'features' ) } className="mobile-background-image" style={ { marginTop: '10px', maxWidth: '100%' } } />
<button
className="button button-large"
onClick={ () => {
setAttributes( { mobileBackgroundImage: '', className: removeClass } );
} }
>
{ __( 'Remove Image', 'features' ) }
</button>
</>
) }
</>
) }
/>
</MediaUploadCheck>
</PanelBody>
</InspectorControls>
</>
);
};
}, 'withClassNameAndInspectorControls' );
addFilter( 'editor.BlockEdit', 'addMobileBackgroundImageControl', withClassNameAndInspectorControls );
/**
* Higher Order Component to add class name to core/group block in the editor.
*
* @param {Function} BlockListBlock - Original component.
* @return {Function} - New component.
*/
const addEditorClass = createHigherOrderComponent( ( BlockListBlock ) => {
return ( props ) => {
const { wrapperProps, name, attributes } = props;
if ( ! [ 'core/group' ].includes( name ) ) {
return <BlockListBlock { ...props } wrapperProps={ wrapperProps } />;
}
const newWrapperProps = {
...wrapperProps,
...( attributes.mobileBackgroundImage &&
{
'data-mobile-background-image': attributes.mobileBackgroundImage,
} ),
};
return <BlockListBlock { ...props } wrapperProps={ newWrapperProps } />;
};
}, 'withTooltip' );
wp.hooks.addFilter( 'editor.BlockListBlock', 'features', addEditorClass );
/**
* Adds the mobileBackgroundImage attribute to the group block settings.
*
* @param {Object} settings - The block settings.
* @return {Object} - The updated block settings.
*/
addFilter( 'blocks.getSaveContent.extraProps', 'addClassNameToGroupBlockFrontend', ( extraProps, blockType, attributes ) => {
if ( attributes?.mobileBackgroundImage ) {
return {
...extraProps,
'data-mobile-background-image': attributes.mobileBackgroundImage,
};
}
return extraProps;
} );
add_filter( 'render_block_core/group', array( $this, 'extend_core_group_block' ), 10, 3 );
public function extend_core_group_block( $block_content, $block, $instance ) {
// Add JavaScript to dynamically set background images.
$image_path="";
if(isset($instance->parsed_block['attrs']['mobileBackgroundImage'])){
$image_path = esc_url( $instance->parsed_block['attrs']['mobileBackgroundImage'] );
}
if(isset($image_path) && $image_path !== ''){
?>
<style>
@media screen and (max-width: 768px){
.wp-block-group.has-mobile-background-image {
background-image: url(<?php echo $image_path; ?>) !important;
background-size: cover;
}
}
</style>
<?php
}
wp_add_inline_script(
'jquery',
'
jQuery(document).ready(function($) {
if ($(window).width() < 768) {
$(".wp-block-group.has-mobile-background-image").each(function() {
var backgroundImageURL = $(this).data("mobile-background-image");
$(this).css("background-image", "url(" + backgroundImageURL + ")");
$(this).css("background-size", "cover");
});
}
});
'
);
return $block_content;
}